Zerynth - custom Python implementation for programming IoT devices
Zerynth is a platform for programming and managing IoT devices integrated with popular cloud platforms. It uses a custom Python implementation that is then compiled for a specific microcontroller.
How does it work?
Zerynth SDK is a set of libraries, IDE and a custom Python implementation that allows programming supported microcontrollers. The Python code is compiled by the IDE and uploaded to a selected board. Python isn't running on the microcontroller like MicroPython. The IDE compiles the code to then run it on Zerynth OS - an RTOS for 32-bit microcontrollers.
Zerynth Device Manager is a web service that allows managing devices that are integrated with the cloud and accessible through the network. Aside of that the company has some other services and features. Generic mobile app to control your boards, 4ZeroBox data acquisition boxes and alike.
The project is commercial in nature and to deploy your production code on multiple microcontrollers you will need appropriate licenses. Zerynth is not oriented on education and makers as CircuitPython, MicroPython, Raspberry Pi and alike. You can however download the IDE and play with your boards without problems. Parts of the project are Open Source.
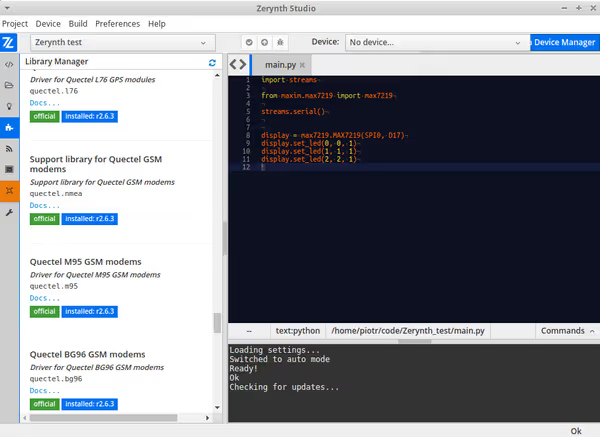
Use cases
Most use cases is centered around IoT - collecting and sending data from sensors, monitoring applications or smart components that can be customized/programmed via Zerynth SDK for end users. Project showcase highlights IoT displays, monitoring of industrial refrigerators, agricultural systems or vehicle tracking and fleet management.
By using Zerynth you are locking yourself to this one platform. Due to how licensing work and capabilities of the platform it doesn't seems as a good choice for mass produced identical devices. It's more tailored to smaller quantities of devices that require customization or more advanced setup (hence all of that monitoring use cases). You can check integrations and use cases pages for more detail.
Working with Zerynth IDE
Zerynth supports multiple microcontrollers and the IDE has additional support for specific boards based on them (like pin layouts). The full list is available in the documentation. Microchip, ESP, STM, Nordic and other vendor microcontrollers are supported.
When working with a board you configure it in the IDE - it installs the Zerynth OS and then handles uploading your compiled code to the board. Installing Zerynth OS wipes existing one so if you would want to use the board with native software stack you may need to re-flash it with original OS.
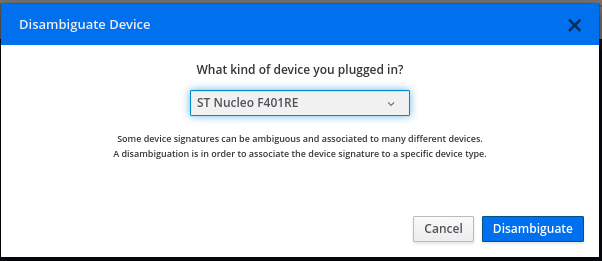

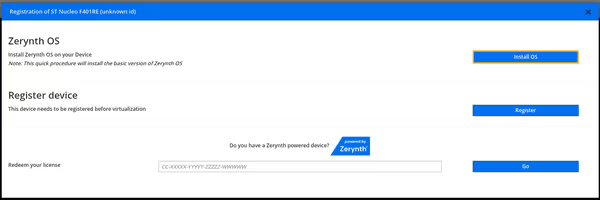
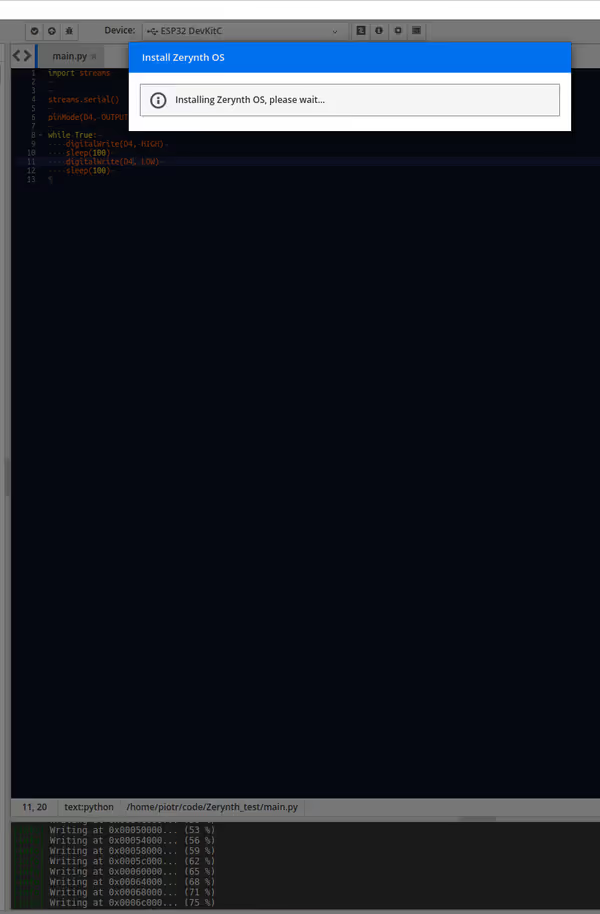
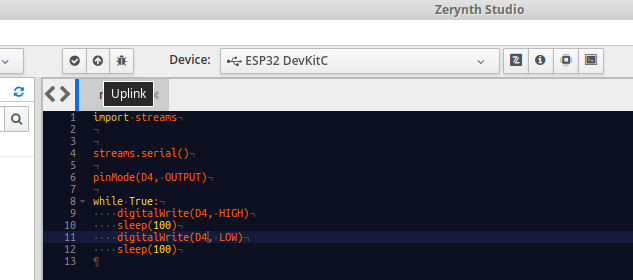
Code examples
To run some examples I used ESP32 Wroom as well as Particle Photon and STM Nucelo boards. ESP configuration and code execution went without any problems. Nucelo board required firmware update but even with that I had problems making it work (old board though). I used Particle Photon board with Zerynth few years ago and the IDE seems to have picked it up although LED hello world did not want to work...
IDE uses a custom Python implementation. Additional modules are installed by the IDE within it software stack. It's not using PyPi nor normal Python implementation.
The boilerplate is super short:
import streams
streams.serial()
# your code here
To blink a LED the code looks like so:
import streams
streams.serial()
pinMode(D4, OUTPUT)
while True:
digitalWrite(D4, HIGH)
sleep(100)
digitalWrite(D4, LOW)
sleep(100)
Where D4
is the digital pin label used in the circuit. Sadly Zerynth uses a lot of global variables/functions.
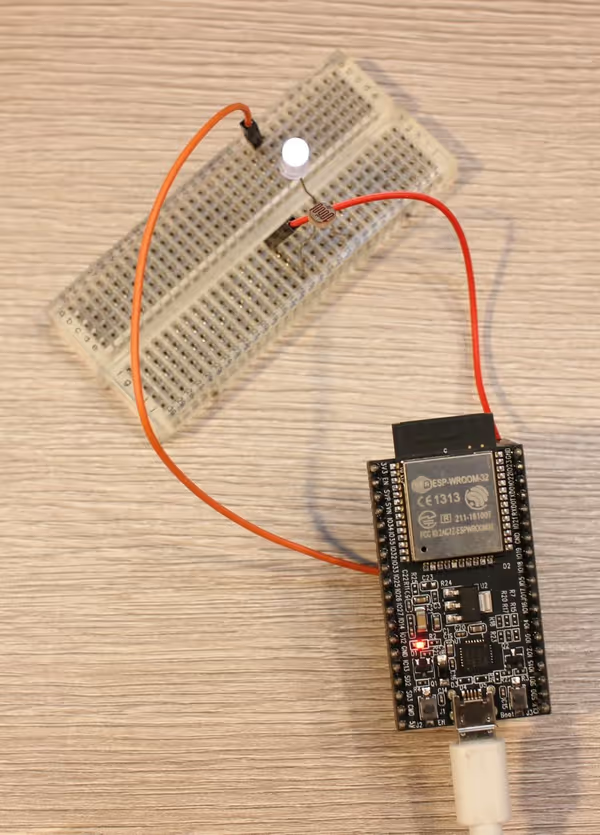
My standard stepper motor (28BYJ-48 stepper motor with ULN2003 controller) code would look like so:
import streams
streams.serial()
pinMode(D4, OUTPUT)
pinMode(D16, OUTPUT)
pinMode(D17, OUTPUT)
pinMode(D5, OUTPUT)
pins = [
D4, D16, D17, D5,
]
steps = [
[D4],
[D4, D16],
[D16],
[D16, D17],
[D17],
[D17, D5],
[D5],
[D5, D4],
]
current_step = 0
def set_pins_low(pins):
[digitalWrite(pin, LOW) for pin in pins]
def set_pins_high(pins):
[digitalWrite(pin, HIGH) for pin in pins]
while True:
high_pins = steps[current_step]
set_pins_low(pins)
set_pins_high(high_pins)
current_step += 1
if current_step == len(steps):
current_step = 0
sleep(2)
As I used ESP32 the pin numbers aren't following an order. First we set pins to output and then we use the digitalWrite
function to set them high and low.
Zeryth has libraries for Neopixels, MAX led arrays and many more. You can browse the list in the IDE and open links to documentation. As this is a custom closed
ecosystem you may face problems if your component is not on the list while has a complex digital interface.
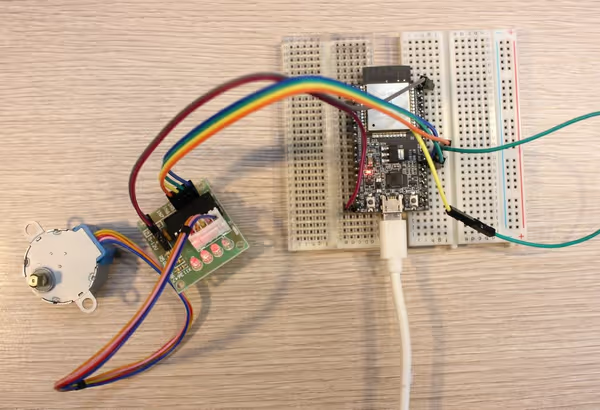
Final Thoughts
I first used Zerynth few years ago. Since then they added more microcontrollers, got more libraries for components but it didn't really picked up in terms of popularity. For one they aren't targeting makers and aren't very active in that group while also focusing more on their commercial side.
The IDE is a really nice tool. Quick access to libraries, pin layout and more. If the board is working then it's easy to program it via Python even if MicroPython or alike aren't available for it. And the code can be more cross-microcontroller compatible than MicroPython.
For existing pro-sumers Zerynth seems to have a sort of a barrier of entry. Instead of going with Atmel, STM or alike and using C code/existing SDK that many developers and companies know and support they would have to vendor-lock the whole project to Zerynth. That's the problem of every self-defined hardware ecosystem like Tinkerforge or Zerynth.
Comment article